Rematch Code Deep Dive: Extra Edition - Dive into Rematch Dispatcher type
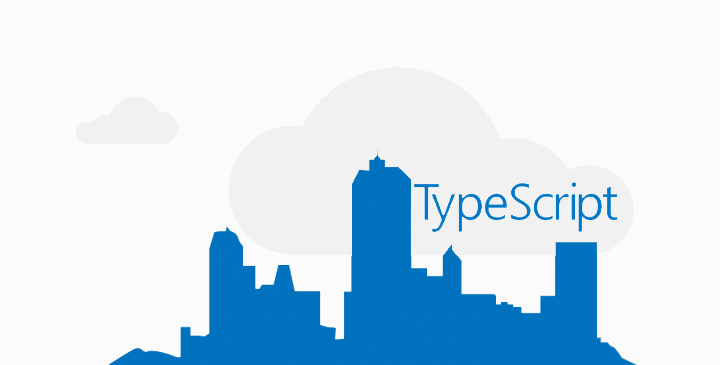
This is Rematch Code Deep Dive Series, an extra edition, discussing the type implementation approach for Rematch Dispatcher.
Recently, I fixed a type related bug in Rematch. Through this bug, I discovered several type bugs still present in Rematch Dispatcher, and there was also redundancy in code organization. Taking this opportunity, I refactored this part and found some interesting aspects.
1 Issue Reproduction
Below is a simple reproduction example, which you can also click to view in the Playground.
|
|
In ExtractDispatcherFromReducer
, I intended to use TRest[0]
to get the payload parameter, and TRest[1]
to get the meta parameter. And I thought when no payload or meta parameter was defined, the corresponding generic parameter in the selected ReducerDispatcher
would be set to the default value void
. However, the reality was different from what I expected; the final parameter turned out to be undefined
. And because [undefined] extends [void] === true
, the effect was the same as [void] extends [void] === true
, so this bug remained undiscovered.
However, once the payload type is any
, this bug could no longer hide. Since [any] extends [void] === true
, it would ultimately enter the () => void
branch, where the payload parameter deduction would be empty, contradicting the definition.
any extends void === true | false
.
So, how to solve this problem? We just need to find a type T
such that [any] extends [T] === false
. Yes, we can use never
to replace void
.
any extends never === true | false
。
That’s where this particular bug is solved. But two problems remain. Firstly, as mentioned earlier, when the meta parameter is not defined, TRest[1]
taken and passed into ReducerDispatcher
is always undefined
, not triggering the default assignment of never
, thus always deducing the parameter to include an optional meta. We can optimize ReducerDispatcher
as follows:
|
|
Due to the addition of a new branch judgment, TRest[1]
is no longer passed into ReducerDispatcher
, allowing TMeta
to be defaulted to never
.
The second problem is that we cannot distinguish between a user-defined type of undefined
and optional parameters. Once a user defines the payload or meta type as undefined | T
(although doing so makes no sense, as the optional symbol ?
could be used directly), the above type gymnastics will fail, appearing as optional parameters even if the user defined undefined | T
type.
Initially, in the design of types, regardless of whether the user defined optional parameters or explicitly undefined
type, the Rematch Dispatcher deduced all as optional parameters after inference. This approach did not lead to runtime errors, and no users have reported related issues. However, this approach is not consistent with the behavior of TS. Out of a desire for continuous improvement, I plan to distinguish between these two this time.
2 Distinguishing Between Undefined
Type and Optional Parameters
Once we can distinguish between a user-defined type of undefined
and the form of optional parameters through gymnastics, we can achieve perfect deduction. The challenge here lies in how to retain the optional nature of parameters while extracting them. Once we use indexing to access specific parameters, their optional nature disappears:
|
|
2.1 Preserving the Array Structure of Optional Parameters
This gave me an idea: if we want to retain the optional nature of parameters, we cannot extract them individually; instead, we must retain the outer array structure:
|
|
Having retained the optional nature of parameters, we then proceed to judge and extract.
2.2 Judging and Extracting Optional Parameters
The following two rules can help us sort out our thoughts:
- Arrays with more parameters cannot be assigned to those with fewer, e.g., the result of
[payload: unknown, meta?: unknown] extends [payload: unknown] ? 1 : 0
will be 0. - Arrays with mandatory parameters can be assigned to those with optional parameters, e.g., the result of
[payload: unknown] extends [payload?: unknown] ? 1 : 0
is 1, but the reverse is not true.
If using Parameters
or infer
to obtain parameter types, the second point above is valid, but if written directly in array form, it is not, as seen in the example below:
|
|
I’m not sure if the above is a bug, and I haven’t found an answer yet. However, since the parameter arrays in Rematch all use infer
for extraction, there is no impact for now.
Using the above logic, we can first judge the situation with fewer parameters (first point), and then continue to deduce the situation with mandatory parameters (second point). Let’s look at the conditional judgments for reducer and effect in Rematch, respectively.
2.3 Extracting Parameters from Reducer
Since we do not need the first parameter state
of the reducer, we first ignore it, then pass all remaining parameters into our newly added type ExtractParametersFromReducer
for processing. As a result, the previously mentioned ExtractDispatcherFromReducer
type can also be simplified, as we pass the parameters to the specialized ExtractParametersFromReducer
for processing, making it very concise.
Additionally, the RematchDispatcher
type in Rematch has also been greatly simplified, as in the previous logic, both RematchDispatcher
and ExtractDispatcherFromReducer
judged parameters, leading to redundancy in the code. Now, the input and output of the ExtractParametersFromReducer
type are both parameter arrays; no preprocessing is required for the input, and the output can be used directly. For details, you can click this PR to view.
Below is the related code:
|
|
Careful readers can see that in ExtractParametersFromReducer
, in addition to infer TPayload
, I also have infer TPayloadMayUndefined
. This is because when a user defines a parameter as p: number | undefined
, the TPayload
type only has number
, and undefined
is ignored. Therefore, here we use TPayloadMayUndefined
to correctly deduce this situation. (The same applies to TMetaMayUndefined
below.)
2.4 Extracting Parameters from Effect
In Rematch’s effects
, the second parameter rootState
is the one we need to ignore. Since it’s not convenient to directly remove the second parameter type, we pass all the parameters to the corresponding ExtractParametersFromEffect
for processing. The code is as follows:
|
|
The situation with effect
is roughly similar to reducer
. In cases containing only payload
, we define the second parameter s
as optional in the condition, so it can hit this branch whether it’s defined or not. Then, for situations with three or more parameters, we first judge for mandatory and finally for optional, consistent with reducer
.
When
effect
was initially designed, considering thatpayload
is a frequently used parameter, it was placed first, withrootState
in the second position. Subsequently addedmeta
was naturally put in the third position, andmeta
is less frequently used. Forreducer
, the currentmodel
’sstate
generally has a higher frequency of access, so it’s placed first, whilepayload
andmeta
are in the second and third positions, respectively.
3 Conclusion
When dealing with such gymnastics problems, it’s important to first think about whether there are constraints that can gradually narrow down the possible range. For example, by the rule that “arrays with more parameters cannot be assigned to those with fewer,” we can use the constraint of “arrays with fewer parameters” to first handle cases with fewer parameters, and then gradually deal with cases with more parameters. Within each case, we continue to use the rule that “arrays with optional parameters cannot be assigned to those with mandatory parameters,” using the constraint of “arrays with mandatory parameters” to first handle mandatory parameter situations.
Also, for multiple parameters, since TS requires optional parameters to be placed after mandatory ones, we prioritize handling the situation where the optional parameters are at the end, and finally deal with the situation where all parameters are optional.
Once you find such patterns, you are a TypeScript magician🏆!